#Topic-2
Last week we have discussed about some basic topics like how to start java , what is the use etc , If you are very new to Java programming then click this link Java Tutorials for Beginners.What is the software’s needed to start JAVA programming.
If you are new to Java programming and wish to learn it right now by doing some hands-on practice, you have visited the right place. This tutorial will help you what is the software’s needed to write your first Java program, Throughout this tutorial, you will learn fundamental concepts and steps which are necessary for every Java fresher.
In order to write and run a Java program, you need below software / editor:
1. Java Development Kit (JDK)
2. Command prompt
3. Note pad
OR
4.IDEs : These IDEs offer a variety of features, like: building Java applications, Testing, debugging, code inspections, code assistance, visual GUI builder and code editor, and more. While you can find several Java IDEs or integrated development environments today, employing the use of the right IDE acts as a powerful software development tool for you. Most popular free Java IDEs like Eclipse and NetBeans and JDeveloper.I prefer Eclipse and we will discuss on later.
First we will write a simple program without using IDEs , here we need JDK tool , Notepad and command prompt.
1. Java Development Kit (JDK)
2. Command prompt
3. Note pad
OR
4.IDEs : These IDEs offer a variety of features, like: building Java applications, Testing, debugging, code inspections, code assistance, visual GUI builder and code editor, and more. While you can find several Java IDEs or integrated development environments today, employing the use of the right IDE acts as a powerful software development tool for you. Most popular free Java IDEs like Eclipse and NetBeans and JDeveloper.I prefer Eclipse and we will discuss on later.
First we will write a simple program without using IDEs , here we need JDK tool , Notepad and command prompt.
1. Java Development Kit (JDK): (Java SE Development Kit). For Java Developers. Includes a complete JRE plus tools for developing, debugging, and monitoring Java applications.
In order to write and run a Java program, you need to install a software program called Java SE Development Kit (or JDK for short, and SE means Standard Edition). Basically, a JDK contains:
- JRE (Java Runtime Environment): is the core of the Java platform that enables running Java programs on your computer. The JRE includes JVM(Java Virtual Machine) that runs Java programs by translating from byte code to platform-dependent code and executes them (Java programs are compiled into an intermediate form called byte code), and other core libraries such as collections, File I/O, networking, etc.
- Tools and libraries that support Java development.
- javac.exe: is Java compiler that translates programs written in Java code into byte code form.
- java.exe: is the Java Virtual Machine launcher that executes byte code.
i.How to download and install JDK:
Click on the following link to download the latest version of JDK installer program:
http://www.oracle.com/technetwork/java/javase/downloads/index-jsp-138363.html
Click on java platform download button as per below screenshot:
Check the option “Accept License Agreement”, and choose an appropriate version for your computer from the list. Here we choose the version for Windows x64:
Verify your computer first , if it is 64 bit then choose Windows x64 as per below screenshot:
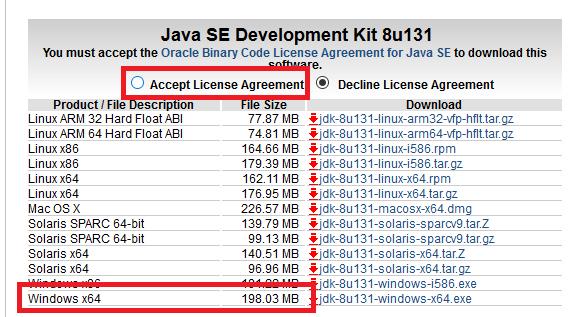
Suppose 32 bit then select Windows x86
After downloading the program, run it and install the JDK on your computer (just following the steps, leave the defaults and click Next, Next, as per below screenshot:
You would see the JDK is installed in the following directory, for example: C:\Program Files\Java\jdk1.8.0_131 The following screenshot describes the JDK’s directory structure:
Now let’s test if Java runtime is installed correctly. Open a command prompt window and type:
java -version
You would see the following result :
That shows version of the JRE, e.g. “1.8.0_131” - Congratulations! Your computer is now capable of running Java programs.
ii. Setting up environment variables or set the class path:
Now we’re going to set environment variables so that the javac.exe program can be accessed anywhere from command line. On Windows 10, go to This PC Properties click - as per below screenshot:
Then click advanced system settings
The System Properties dialog appears select advanced tab and click Environment Variables, as per below screenshot:
The Environment Variable dialog appears, click on the New button under the System variables section, as per below screenshot:
The field Variable name must be JAVA_HOME, and the field Variable value must point to JDK’s installation directory on your computer. Here it is set to C:\Program Files\Java\jdk1.8.0_131. Click OK to close this dialog.
Now back to the Environment Variables dialog, look for a variable called Path under the System Variables list, and click Edit.
In the Edit System Variable dialog, append the following to the end of the field Variable value: ;%JAVA_HOME%\bin
OR
If you can’t append the value then click to edit again click New add ;%JAVA_HOME%\bin (Windows 10)
Note that there is a semicolon at the beginning to separate this value from other ones. Click OK three times to close all the dialogs.
Now let’s test if Java class path set correctly or not. Open a command prompt window and type: javac -version
You would see the following output:
Congratulations! You have completed the setup for essential Java development environment on your computer. It’s now ready to write your first Java program.
iii. Writing a Java hello world program
Open a simple text editor program such as Notepad and type the following content:
Save the file as HelloWorld.java (note that the extension should be .java) under a directory, let’s say, E:\Java. [Select save as type : All files]
Don’t worry if you don’t understand everything in this simple Java code. Please note following descriptions:
Let's go over the Hello world program, which simply prints "Hello, World!" to the screen.
The first line defines a class called HelloWorld.
public class HelloWorld {
In Java, every line of code that can actually run needs to be inside a class. This line declares a class named HelloWorld, which is public, that means that any other class can access it. This is not important for now, so don't worry. For now, we'll just write our code in a class called HelloWorld, and talk about objects later on.
Notice that when we declare a public class, we must declare it inside a file with the same name (HelloWorld.java), otherwise we'll get an error when compiling.
The next line is:
public static void main(String[] args) {
This is the entry point of our Java program. The main method has to have this exact signature in order to be able to run our program.
- public again means that anyone can access it.
- static means that you can run this method without creating an instance of HelloWorld.
- void means that this method doesn't return any value.
- main is the name of the method.
The next line is:
System.out.println("Hello world!");
System is a pre-defined class that Java provides us and it holds some useful methods and variables.
out is a static variable within System that represents the output of your program (stdout).
println is a method of out that can be used to print a line.
iv. Compiling it
Now let’s compile our first program in the HelloWorld.java file using javac tool. Type the following command to change the current directory to the one where the source file is stored:
E:\Java
And type the following command:
javac HelloWorld.java
That invokes the Java compiler to compile code in the HelloWorld.java file into byte code. Note that the file name ends with .java extension. You would see the following output:
If everything is fine (e.g. no error), the Java compiler quits silently, no fuss. After compiling, it generates the HelloWorld.class file which is byte code form of the HelloWorld.java file.
Go to file directory you can see HelloWorld.class file :
V. Running it
It’s now ready to run our first Java program. Type the following command:
java HelloWorld
That invokes the Java Virtual Machine to run the program called HelloWorld (note that there is no .java or .class extension). You would see the following output:
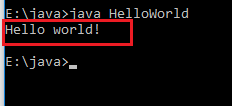
It just prints out “Hello world!” to the screen and quits. Congratulations! You have successfully run your first Java program!
Next week I will post about(#Topic-3) How to Install Eclipse and Get Started with Java Programming
If you enjoyed this post, I’d be very grateful if you’d help it spread by emailing it to a friend, or sharing it on Twitter or Facebook. Thank you! - Shivananda Rai
easy to understand , step by step detail make easy to understand
ReplyDelete